Overview
CoinBook is a desktop Cryptocurrency management app for storing and keeping track of one’s cryptocurrency portfolio. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is a Java application targeted at enthusiasts or investors who trade heavily and actively in cryptocurrencies. It provides users a single platform on which they can check news and statistics along with managing their cryptocurrency portfolio.
Summary of contributions
-
Major enhancement: added the ability to sort Coin List
-
What it does: allows the user to sort list of coins by lexicographical order.
-
Justification: This feature improves the product significantly because a user can make arrange his coins in such a way that is easier for him to view and find the desired coin he wants to interact with, especially if said user has a diverse and big portfolio to deal with.
-
Highlights: This enhancement does not affect any existing commands and is a standalone feature. It did not require in-depth analysis however the implementation was relatively challenging as some understanding of the inter-link between the ParserUtil, CoinBookParser, ModelManager and Model classes was essential in figuring out how to eventually make the SortCommand function come to fruition.
-
Credits: Required some help to search other implementations of other sort functions in order to understand how to apply it for our own CoinBook
-
-
Minor enhancement: added an additional option that allows for sorting by reverse alphabetical order by adding another case.
-
Code contributed: [Functional code] [Test code]
-
Other contributions:
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Change listing order order
| o
[Since v1.5]
order {a,z}
Orders the coin listing in lexicographical order or reverse lexicographical order.
Put a
after the command word to sort it in lexicographical order, and z
to sort in reverse lexicographical order. The listing is sorted by the leftmost entered option first, then equal values are sorted by the next one, and so on.
The default order is lexicographical order of the coin names (i.e., option a
).
order a
Sort the listing in lexicographical order (default option, whether "a" is added after the command word or not).
order z
Sort the listing in reverse lexicographical order.
This command can be reversed with |
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Organisation Features
Sorting
The sorting mechanism is facilitated by Collections.sort
. It supports sorting the coin list passed into the sort method to arrange the coins in a manner that the user chooses.
The way Collections.sort
works is that it takes the collection’s underlying array and calls its sort method to sort the actual elements. The sorting algorithm used by Java is Timsort.
This sorts the collection in-place, i.e., it modifies the given collection by sorting its elements directly. As a result, a sorted copy need not be made, saving resources.
The diagram (Fig. 25) below is the Sequence Diagram for interactions that occur from when the sort command is first called by the user to when the Coin List is sorted.
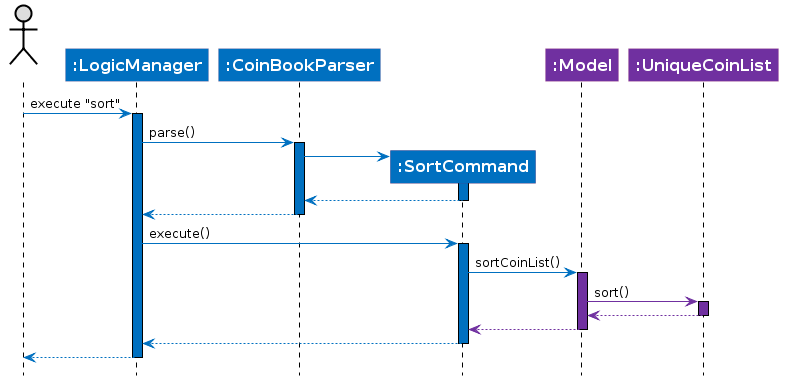
The order mechanism is facilitated by SortCommand
which is also an UndoableCommand
.
SortCommandParser
checks for the sort order (a or z parameter) to decide the relevant SortCommand
to call.
The internalList
object is then sorted using Java’s sort method.
How the sort procedure works:
-
Sort by lexicographical order
-
We can use
compareTo()
for comparing coin code names. For CoinBook, we want to order our list of coins lexicographically, based on the alphabetical order of their component letters. This is done by passinginternalList
into the sort function which then usesgetCode()
to compare each of the component letters of the coin code, which will then arrange coins based on the lexicographical value of the code string. The coin code strings with the lowest lexicographical value will appear first followed by coin code strings with higher lexicographical values as you go further down the list. -
We can either use the
reversed
function onComparator
or change the compare condition ofcompareTo()
to invert the default setting and arrange the coins from highest to lowest lexicographical value instead. -
We do not have to pass in any comparator for the list to be correctly sorted because the 3-letter coin code is a
String
which implements theComparable
interface, and hence guarantees its own implementation of the sort comparator.
-
Grouping [Coming in v2.0]
The group feature can use the Collectors
class, which provides methods for grouping data stored in collections. Grouping would permit the user to organise coin data based on a common field, e.g coins prices equal to or over 1000 dollars per coin.
The GroupingBy
method from the Collectors
class is responsible for organising the coin data into groups. In this case the group is defined by the value of the coin. The Collect
method from the Stream
class accepts the GroupingBy
method as its argument and returns a map containing the results. The results are then displayed.
Using the GroupingBy
collector from the Collector
class, it takes a single parameter (or classifier) that assigns a grouping key to every stream element.
By default, elements with the same key are inserted into a List<T>
, although this can be changed by specifying a second parameter to GroupingBy
.
Design Considerations
Aspect: Implementation of SortCommand
-
Alternative 1 (current choice): Use
Collections.sort
-
Pros: There is no need to pass any comparator for the coin list since
String
already implements theComparable
interface. -
Cons: The developer needs to understand the relationship between array lists and collections.
-
-
Alternative 2: Add a new sorting function (e.g. Selection Sort)
-
Pros: This possibly allows us to reduce the time required to execute the sorting command.
-
Cons: This would take more time and a considerable amount of effort to incorporate into our CoinBook since we would not be using Java’s in-built sorting method.
-
Aspect: Undo-ability of SortCommand
-
Alternative 1 (current choice): Make it an
UndoableCommand
just likeadd
,clear
,edit
etc.-
Pros: This offers an intuitive, single interface for similar operations.
-
Cons: This would increase the coupling between the coin data and the rule data parts of
Model
, since the current implementation ofUndoableCommand
requires saving the state ofModel
, which is a wrapper for just the coin data.
We want to keepModel
as an interface for just the coin data itself.
-
-
Alternative 2: Leave it non-undoable
-
Pros: Sort Command data can be kept separate from coin data.
-
Cons: The UI will be less intuitive as users would have to change how they manage coins and rules.
The user would not be able to undo the sort order once it is applied, and this might be inconvenient if the user wanted the sort function for a temporary means only or the user accidentally triggered the sort.
Sometimes a user might want their own customised way of arranging coins and so the non-undoable nature might compromise this.
-
Aspect: Implementation of Groups function [Coming in v2.0]
-
Alternative 1 (current choice): Use
GroupingBy
method-
Pros: The user has an additional option where he can view coins based on a specified attribute.
-
Cons: The developer needs to understand the relationship between
Stream
,ArrayList
andCollector
classes.
-
-
Alternative 2: Add additional tags in lieu of grouping
-
Pros: The codebase already has support for tags and so not a lot of changes will be required.
-
Cons: Commands will not be executable at a group level because tags merely depict the attribute of the coins, but groups act as a tool to manage several coins at the same time. I.e.: Grouping would add more functionality to the CoinBook for mass coin management.
-