Overview
CoinBook is a desktop accounting application written in Java. It is targeted at cryptocurrency traders and enthusiasts, and allows them to keep track of the coins they hold, obtain price data and analytics, and read the latest news relevant to them in the same place. Primary interaction is through a CLI, and a GUI built with JavaFX.
Summary of contributions
-
Major enhancement: added a sync command that updates all price-related metrics of cryptocurrencies with latest, live data
-
What it does: allows the user to keep up to date with the latest price movements of cryptocurrencies invested.
-
Justification: This feature improves the product significantly because the volatile market that cryptocurrencies operate in necessitates that a user is frequently kept up to date with all changes in cryptocurrency prices.
-
Highlights: This enhancement demonstrates live updating of data from external web APIs. It required analysis of design alternatives between synchronous and asynchronous data fetching. The implementation too was challenging as it required many utilities to be built such as UrlBuilderUtil to adhere to HTTP request formats and FetchUtil to perform API calls.
-
Credits: External libraries used include: [Apache HttpClient], [GSON] and [AsyncHttpClient]
-
-
Minor enhancement: added a autocomplete feature that allows users to quickly enter in commands.
-
Code contributed: [Functional code] [Test code]
-
Other contributions:
-
Project management:
-
Managed releases
v1.1
(1 release) on GitHub
-
-
Enhancements to existing features:
-
Improve GUI by adding coin icons to each coin panel (Pull requests #233)
-
Improve UX by overlaying a loading animation when asynchronous operations are carried out (Pull requests #241)
-
Add API for backup storage (Pull requests #17)
-
Refactored existing tests for existing features to suit new code base (Pull requests #110)
-
-
Documentation:
-
Enable experimental attributes such as keyboard macros for the Developer Guide: #124
-
-
Community:
-
Tools:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Sync with price server sync
| sy
[Since v1.5]
sync
Obtains the latest price data to update price metrics of all owned coins.
You may notice some coins disappearing from the list view after syncing. This is most likely because the relevant coins no longer satisfy the currently applied list filter. Run the list command if you wish to see all coins.
|
Autocomplete [Since v1.5]
Pressing any key will auto-suggest the field with all possible matching inputs.
Pressing the UP and DOWN arrow keys will allow navigation of the input suggestions.
Once the desired command is selected as indicated by light grey highlight, pressing the ENTER key will input the command into the command box.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Sync Feature
The sync mechanism is facilitated by FetchUtil
, which uses a 3rd party library AsyncHttpClient
that supports asynchronous dispatching of HTTP requests.
Suppose the user wants to update the prices of all coins owned with the latest data.
The user executes the sync command using SyncCommand
, i.e. sync
, which first builds the API URL. The API URL is built using UrlBuilderUtil
, which first retrieves the coin code of every coin account that the user has, then concatenates to CryptoCompare API URL as HTTP parameters.
FetchUtil
is then used to send a HTTP GET request to CryptoCompare using this built URL via AsyncHTTPClient
and returns a Future<Response>
to SyncCommand
. When the response data from the Future<Response>
object is received, this data is then deserialized into Price
objects to be passed to the ModelManager
for updating.
Once the ModelManager
receives the new Price
objects, it passes them to the CoinBook
to carry out price updates for each coin via CoinChangedEvent
.
The following sequence diagram (Fig. 26) is a visual representation of the above described process.
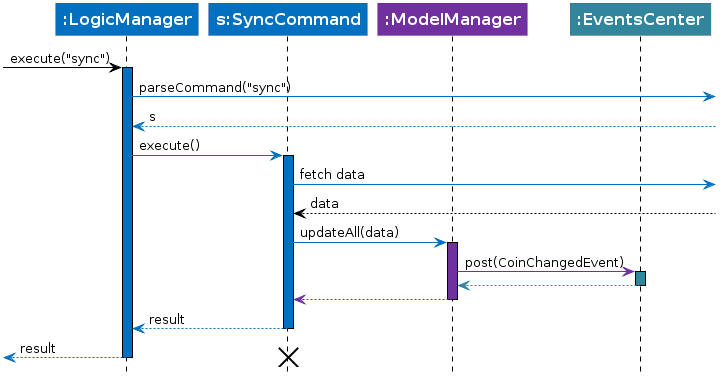
Design Considerations
Aspect: Asynchronous vs synchronous fetching
-
Alternative 1 (current choice): Asynchronous fetching
-
Pros: This allows background processes to still continue, such as a loading animation to provide visual feedback to the user that data fetching is in progress and the application has not hanged.
-
Cons: This is more tedious to implement and requires the use of 3rd party libraries that may have bugs.
-
-
Alternative 2: Synchronous fetching.
-
Pros: This is easier to implement and only uses Java API which is much more robust and less susceptible to unforeseen bugs.
-
Cons: This leads to a worse user experience as the user may not know if the sync command is actually running or the application has hanged.
-
Autocomplete Feature
The autocomplete mechanism is facilitated by CommandBox
, which uses COMMAND_LIST
from CommandList
. It supports the predicting of a word or phrase that the user may type based on a partial text query.
Suppose the user is trying to use the sell
command.
The user will first type s
into the command line.
s
Now, the autocomplete feature will then auto-suggest inputs to complete the query by displaying a pop-up of all commands that contain the letter s
(See Fig. 27).
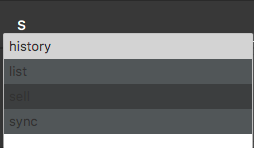
When the user then presses DOWN twice to reach his desired sell
command, the selected suggestion will be highlighted light grey (See Fig. 28).
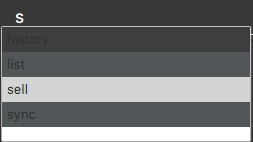
The user can then press Enter to confirm his selection of the sell
command.
sell
Design Considerations
Aspect: The fields which autocomplete works on
-
Alternative 1 (current choice): Apply only for command word
-
Pros: This reduces the implementation cost.
-
Cons: The reduced functionality may not satisfy every user.
-
-
Alternative 2: Apply for both command word and command parameters.
-
Pros: User experience will be slightly improved over the alternative.
-
Cons: This has a lower value to effort ratio to implement due to restrictions of the ControlsFX and JavaFX libraries.
-
Aspect: Data structure to store the list of commands
-
Alternative 1 : Use a Radix Tree
-
Pros: This is easier to understand for new Computer Science undergraduates, who represent the majority of incoming developers to the project.
-
Cons: The implementation could be non-trivial.
-
-
Alternative 2 (current choice): Use a linear data structure
-
Pros: This is much easier to implement and integrates nicely with ControlsFX.
-
Cons: The solution will be inefficient, with a higher time complexity incurred.
-